Order Callback - NodeJS Example
Below is a NodeJS example of using the Subscribe Pro Order Callback feature.
For this example, we've set up an Express server to act as our e-commerce platform and handle order placement requests sent from Subscribe Pro.
const express = require('express');
const router = express.Router();
const crypto = require('crypto');
// The shared secret entered in Subscribe Pro:
const SHARED_SECRET = 'yoursecretkey';
// Middleware to authenticate using SHA-256 HMAC algorithm
const authValidate = function (req, res, next) {
// Get the HMAC header
const spHmac = req.get('Sp-Hmac');
// Throw a 403 error if the header doesn't exist
if (!spHmac) {
res.status(403).json({
Error: 'Unauthorized request',
});
}
// Use SHA-256 HMAC algorithm to generate hash
const hmac = crypto.createHmac('sha256', SHARED_SECRET);
data = hmac.update(JSON.stringify(req.body));
// Check to be sure the hash signatures match
if (spHmac === data.digest('hex')) {
next();
} else {
res.status(403).json({
Error: 'Unauthorized request',
});
}
};
// Place order on the e-commerce platform using the request data
const placeOrder = function (req, res, next) {
const orderRequest = req.body;
console.log('Placing order...');
// ...
console.log('Order has been placed.');
next();
};
// Send back a POST request back to Subscribe Pro with order information
router.post('/', authValidate, placeOrder, function (req, res) {
// Build order details
const order = {
orderNumber: 'ABC-091293923',
orderDetails: {
customerId: '123456',
customerEmail: '[email protected]',
platformCustomerId: '1234',
platformOrderId: '091293923',
orderNumber: 'ABC-091293923',
salesOrderToken: '89adsfjkl129834kljsad98fuoi123uj489u23894',
orderStatus: 'placed',
orderState: 'open',
orderDateTime: '2021-09-16T17:43:00Z',
currency: 'USD',
shippingTotal: '7.2000',
taxTotal: '4.0000',
total: '51.2000',
billingAddress: {
firstName: 'Joe',
lastName: 'Customer',
},
shippingAddress: {
firstName: 'Joe',
lastName: 'Customer',
},
items: [
{
platformOrderItemId: '735641231234',
productSku: 'test-product',
productName: 'Test Product',
shortDescription: 'Subscribe Pro Test Product',
qty: '1',
requiresShipping: true,
unitPrice: '25.0000',
shippingTotal: '7.2000',
taxTotal: '4.0000',
lineTotal: '36.2000',
subscriptionId: '543210',
},
],
},
};
// Send back the JSON order details with a 201 status code
res.status(201).json(order);
});
module.exports = router;
Example Usage
In the following example, we will test recurring order placement using the Order Callback feature and the code above.
- In the Subscribe Pro Merchant App, we'll first configure our Order Callback credentials in System > Configuration > Store Connection Settings. (We're using ngrok to access our local Express server publicly.)
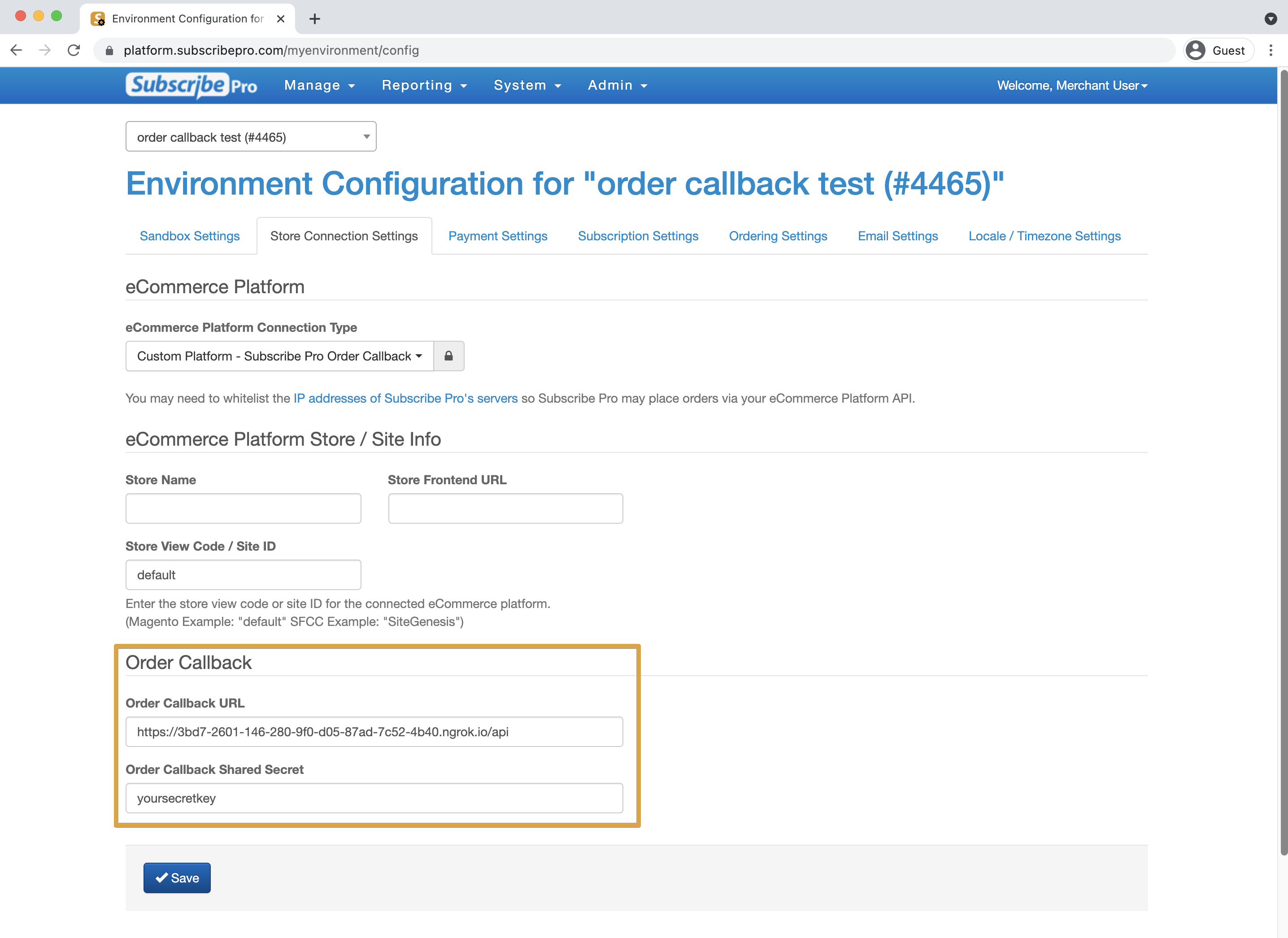
- Next, we will go to Manage > Customers, choose a sample customer, and click Edit.
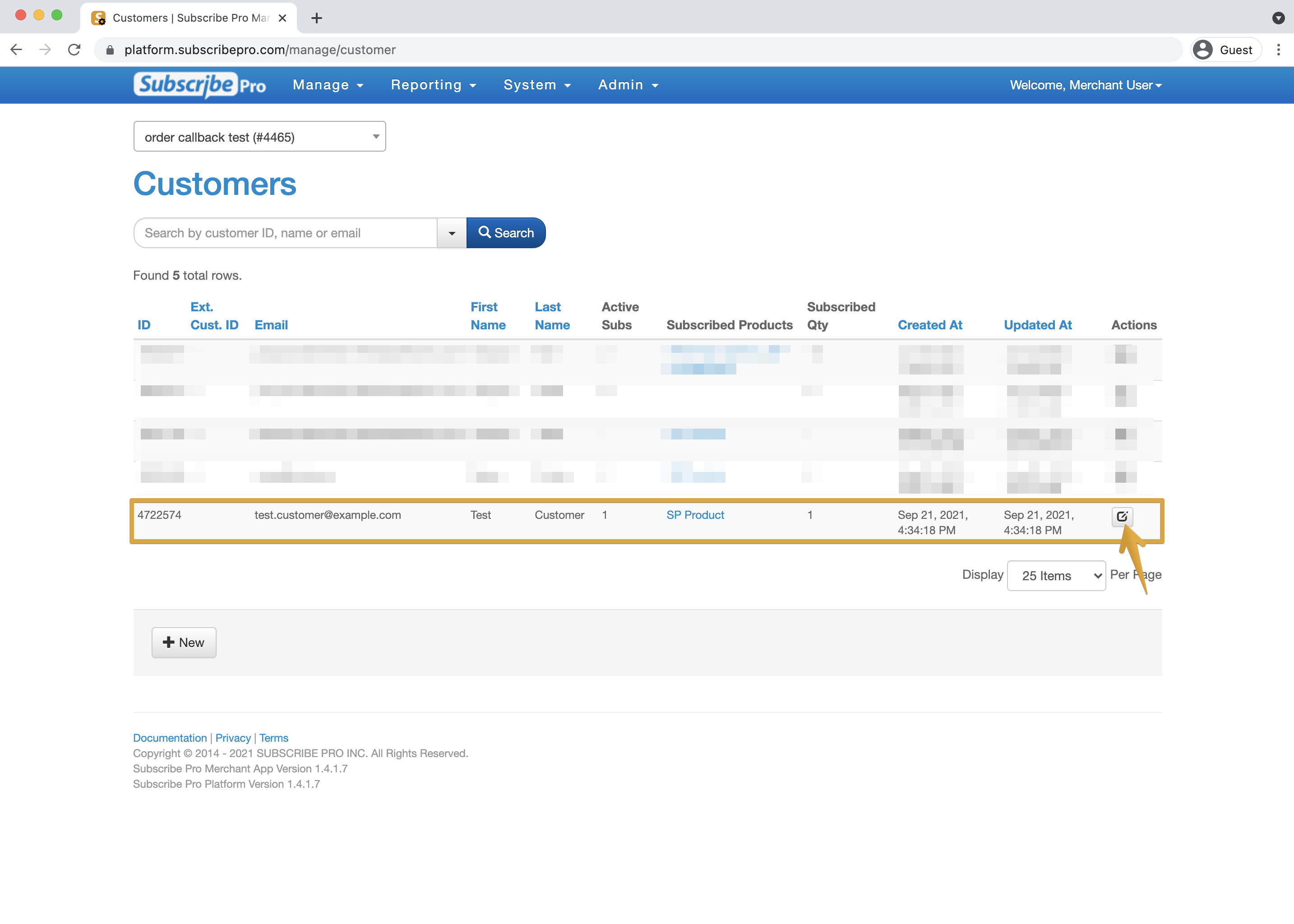
- Then, we'll find a sample subscription to use and manually place the recurring order by clicking Place Order, selecting "Recurring Order," and clicking the "Place Order" button.
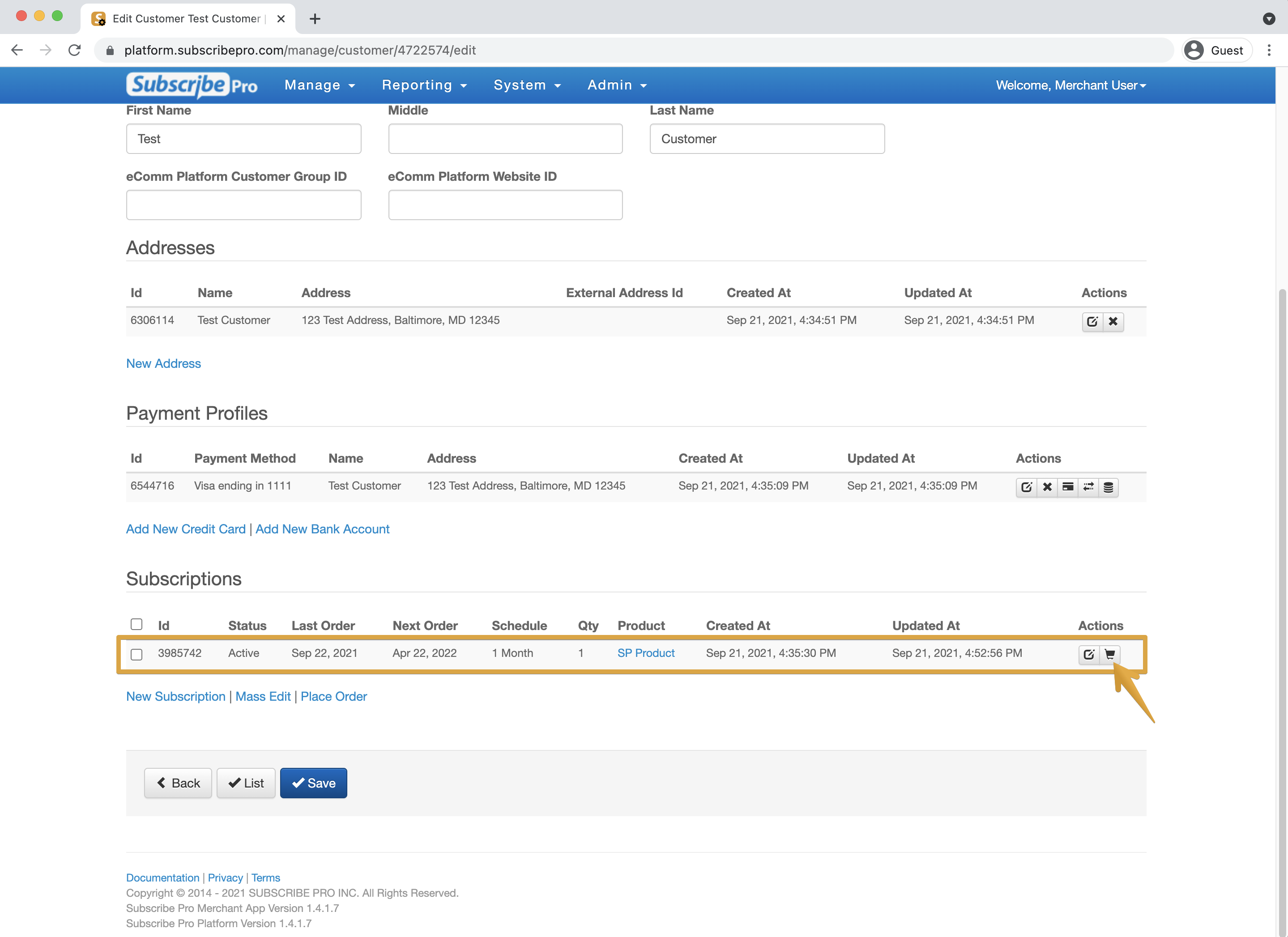
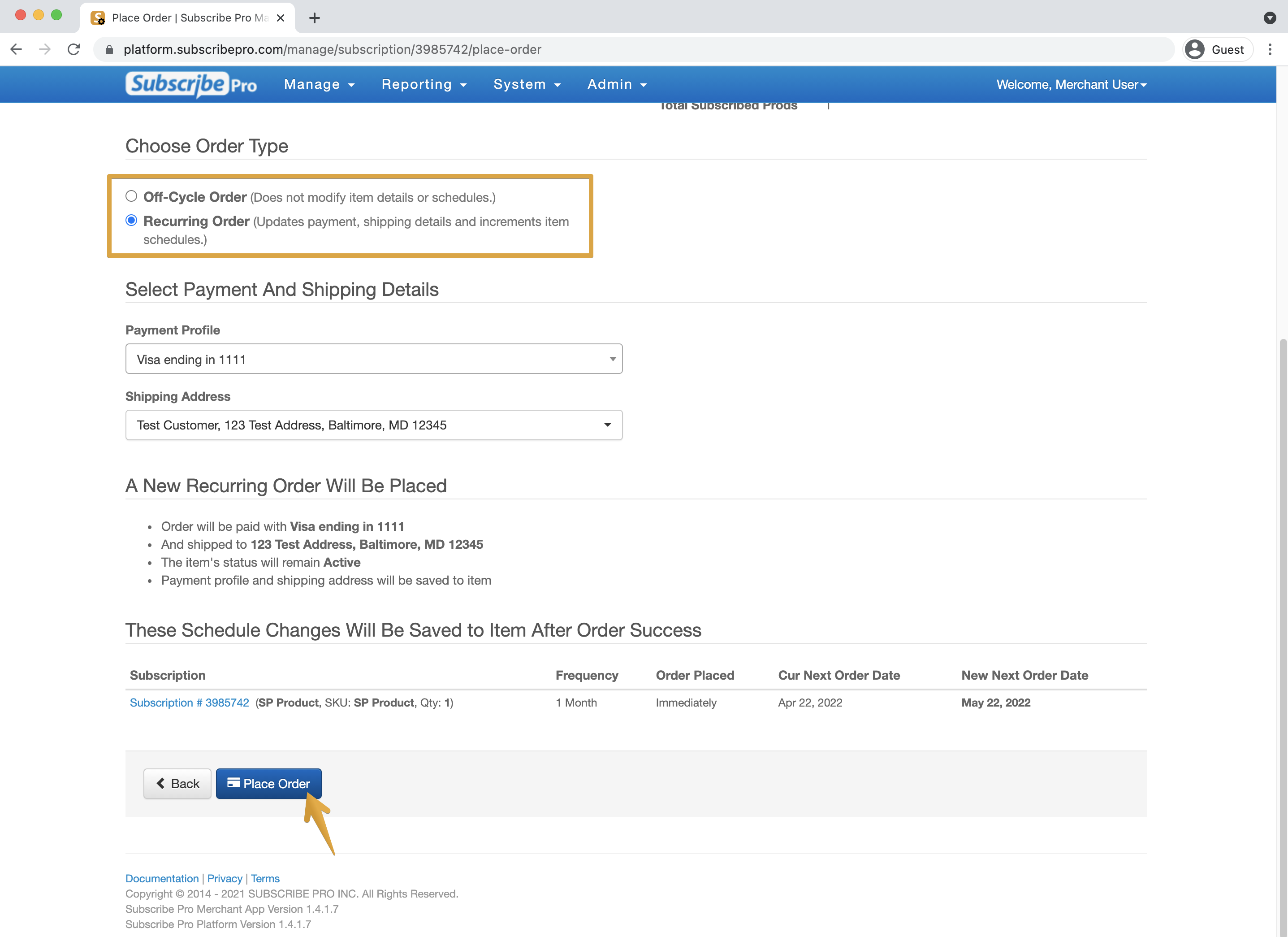
- The subscription is now queued up and will process with the next batch of subscriptions in a couple of minutes. Once the order has completed, you should see the successful order in Subscribe Pro.
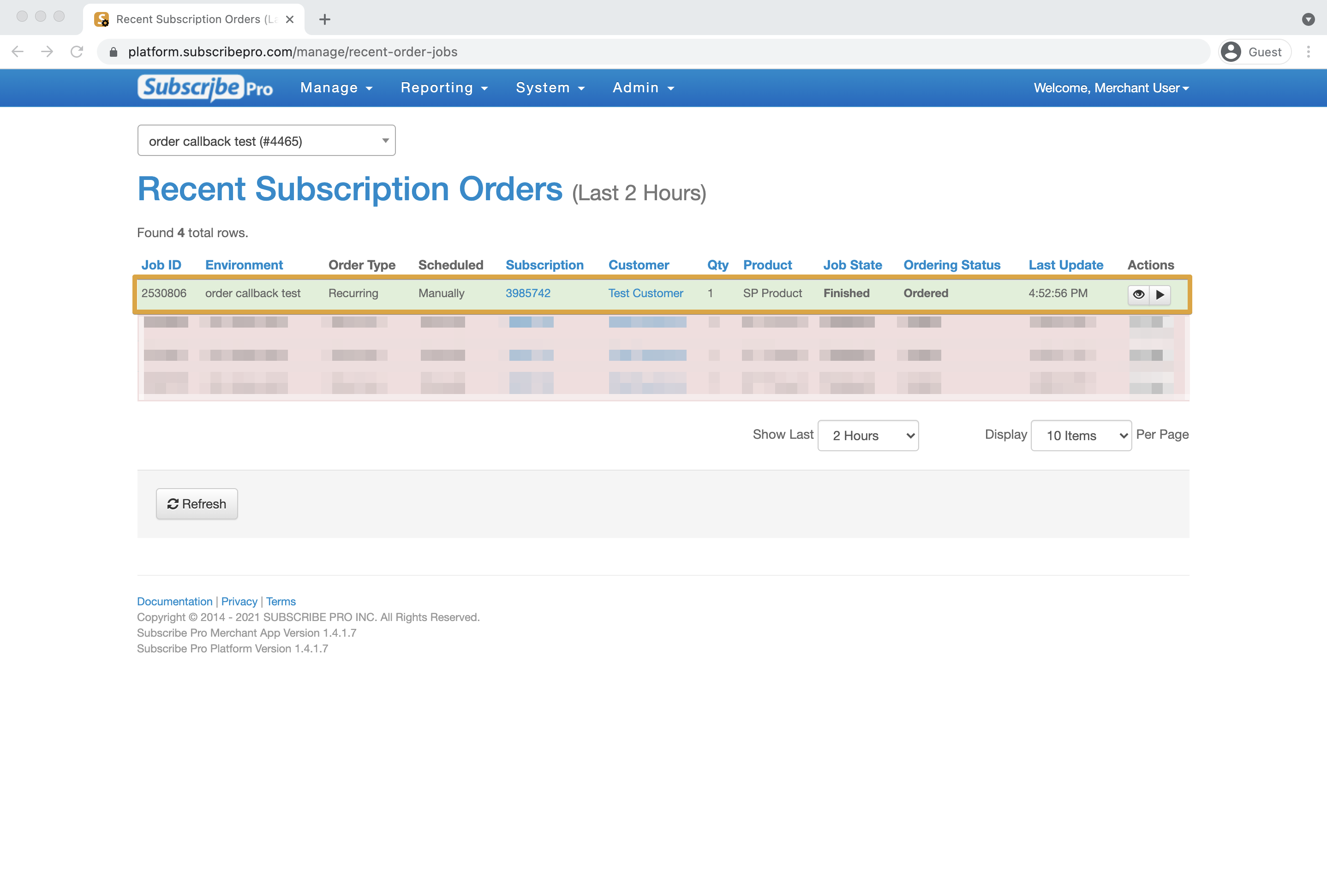