Webhook Security
Subscribe Pro sends webhook notifications for many different events. To ensure maximum security and validate that the webhooks you are receiving were generated by Subscribe Pro's subscription commerce platform, you should implement the following measures.
Validate Webhook Request Signatures
The webhooks Subscribe Pro sends to your system will contain a secure hash signature in the Sp-Hmac
header. The hash is generated using a keyed SHA-256 HMAC algorithm.
Find The Shared Secret
The secret key used in generating the secure hash for your webhooks can be found in the Subscribe Pro Merchant App. Each webhook destination has its own unique Shared Secret.
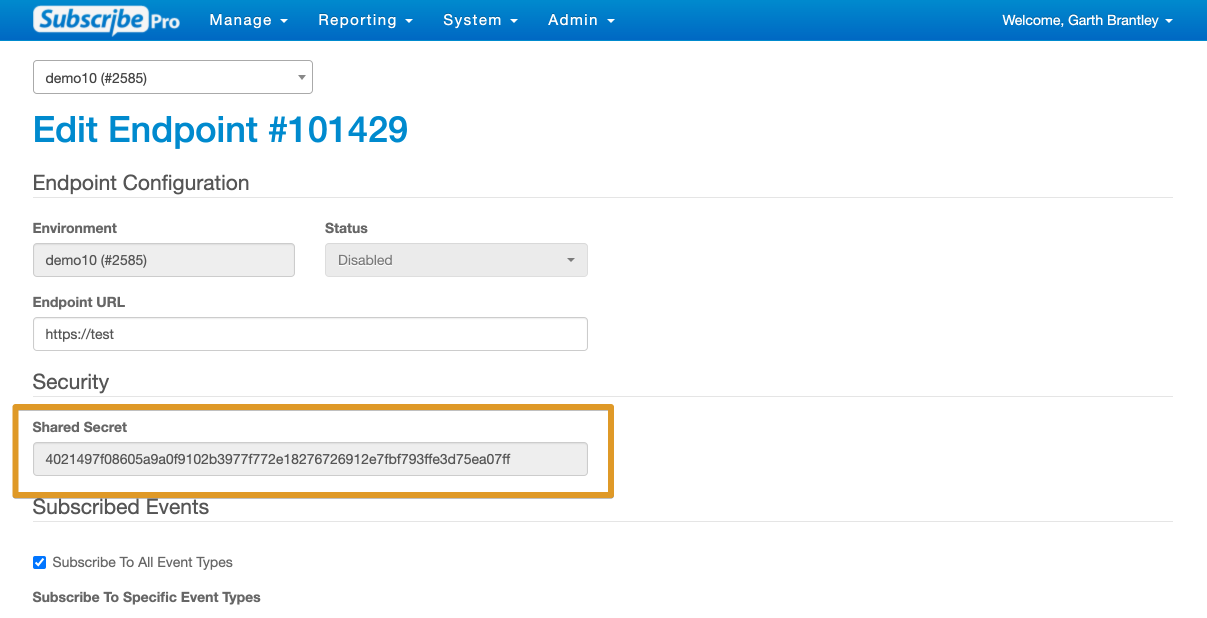
Validate The Signature
The following is an example of how to validate the signature with PHP code:
class WebhookValidatorExample
{
const SP_HMAC_HEADER = 'Sp-Hmac';
/**
* @param \GuzzleHttp\Psr7\Request $request
* @param string $sharedSecret
*
* @return bool
*/
public function validateRequestHmac(\GuzzleHttp\Psr7\Request $request, $sharedSecret)
{
// Get signature from request header
$hmacSignature = $request->getHeader(self::SP_HMAC_HEADER);
// Get request body (JSON string)
$body = $request->getBody();
// Calculate the hash of body using shared secret and SHA-256 algorithm
$calculatedHash = hash_hmac('sha256', $body, $sharedSecret, false);
// Compare signature using secure compare method
return hash_equals($calculatedHash, $hmacSignature);
}
}
Whitelist Subscribe Pro IPs
You should block all IP addresses and explicitly whitelist all of the IP addresses listed under Subscribe Pro Commerce Platform here:
Retrieve Event Data From Subscribe Pro API
We include detailed webhook event data in the webhook message body as a convenience. However to ensure security, and validate that the webhook data you received was generated by Subscribe Pro, you should make a call back to our API to receive the webhook event data before processing.
An example webhook event request body (v2) looks like the following:
{
"webhook_event": "{\"id\":98989898, ... \"}",
"webhook_event_url": "//services/v2/webhook-events/98989898"
}
To call the Subscribe Pro API and thus validate the webhook event data you are using, use the webhook_event.id
and/or webhook_event_url
properties from the webhook message body and make a call to the /services/v2/webhook-events/{id} API endpoint.
An example curl request would look like this:
curl -X GET https://api.subscribepro.com/services/v2/webhook-events/98989898 \
-H "Authorization: Bearer ACCESS_TOKEN_GOES_HERE" \
-H "Content-Type: application/json"