HTTP Order Callback Security
To secure your order callback endpoint, your handler must validate that the request has come from Subscribe Pro. There are a few different approaches to handle this.
Validate Callback Request Signatures
The HTTP callbacks Subscribe Pro sends to your system will contain a secure hash signature in the Sp-Hmac
header. The hash is generated using a keyed SHA-256 HMAC algorithm.
Find The Shared Secret
The secret key used in generating the secure hash for your callback requests can be found in the Subscribe Pro Merchant App.
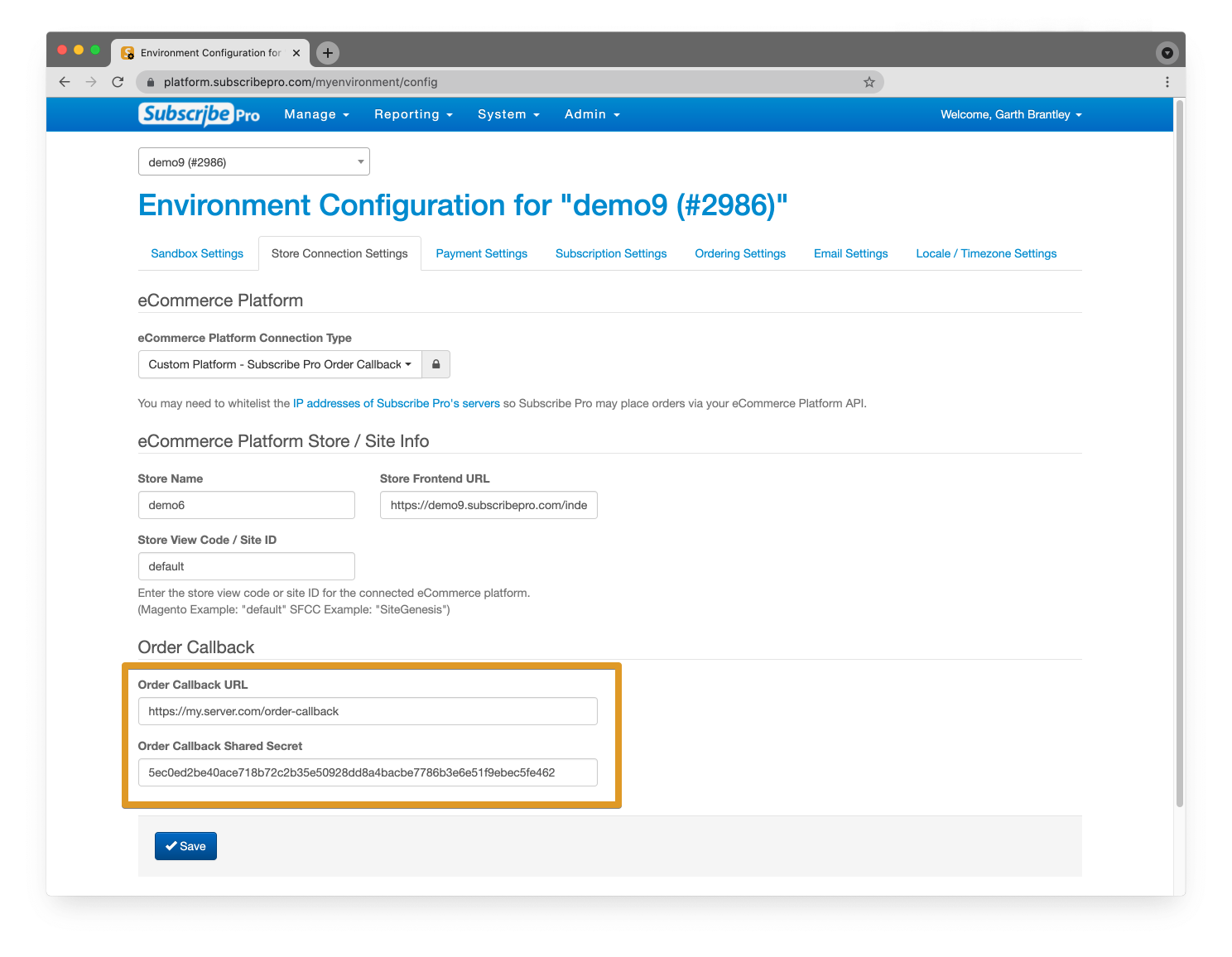
Validate The Signature
The following is an example of how to validate the signature with PHP code:
class CallbackSignatureValidatorExample
{
const SP_HMAC_HEADER = 'Sp-Hmac';
/**
* @param \GuzzleHttp\Psr7\Request $request
* @param string $sharedSecret
*
* @return bool
*/
public function validateRequestHmac(\GuzzleHttp\Psr7\Request $request, $sharedSecret)
{
// Get signature from request header
$hmacSignature = $request->getHeader(self::SP_HMAC_HEADER);
// Get request body (JSON string)
$body = $request->getBody();
// Calculate the hash of body using shared secret and SHA-256 algorithm
$calculatedHash = hash_hmac('sha256', $body, $sharedSecret, false);
// Compare signature using secure compare method
return hash_equals($calculatedHash, $hmacSignature);
}
}
Whitelist Subscribe Pro IPs
You should block all IP addresses and explicitly whitelist all of the IP addresses listed under Subscribe Pro Commerce Platform here: